顺序栈
1. 顺序栈定义
栈(Stack):是只允许在一端进行插入或删除的线性表。首先栈是一种线性表,但限定这种线性表只能在某一端进行插入和删除操作。
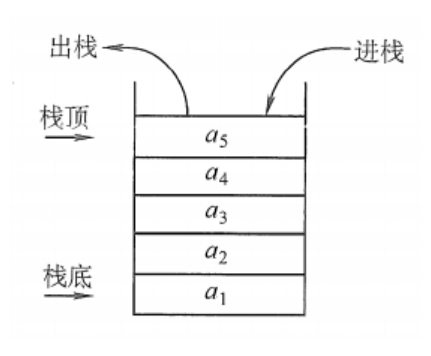
栈又称为后进先出(Last In First Out)的线性表。
1 2 3 4 5 6
| typedef struct Stack { STDataType* a; int top; //有效数据个数 int capacity; //容量 }ST;
|
2. 顺序栈初始化
1 2 3 4 5 6
| void StackInit(ST* ps){ assert(ps); ps->a = NULL; ps->capacity = 0; ps->top = 0; };
|
3. 顺序栈销毁
1 2 3 4 5 6 7
| void StackDestroy(ST* ps){ assert(ps); free(ps->a); ps->a = NULL; ps->capacity = 0; ps->top = 0; };
|
4. 顺序栈插入(压栈)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| void StackPush(ST* ps,STDataType x){ assert(ps); if(ps->capacity == ps->top){ //判断容量是否足够,如果不够则增容 int newcapacity = ps->capacity ==0?4:(ps->capacity)*2; STDataType* temp = (STDataType*)realloc(ps->a,sizeof(STDataType)*newcapacity);//扩容 if(temp == NULL){ printf("realloc fault"); exit(-1); } ps->a = temp; //把扩容地址传给a ps->capacity = newcapacity; } ps->a[ps->top] = x; //插入元素x ps->top++;
};
|
5. 顺序栈删除(出栈)
1 2 3 4 5
| void StackPop(ST* ps){ assert(ps); assert(StackEmpty(ps) != 1); ps->top--; };
|
6. 栈顶元素查看
1 2 3 4 5 6
| STDataType StackTop(ST* ps){ assert(ps); assert(StackEmpty(ps) != 1);
return ps->a[ps->top-1]; };
|
7. 查看栈的元素个数
1 2 3 4 5
| int StackSize(ST* ps){ assert(ps); return ps->top; };
|
8.判断栈是否为空 为空返回1
1 2 3 4 5 6 7 8
| int StackEmpty(ST* ps){ assert(ps); if(ps->top == 0){ return 1; } else return 0; };
|